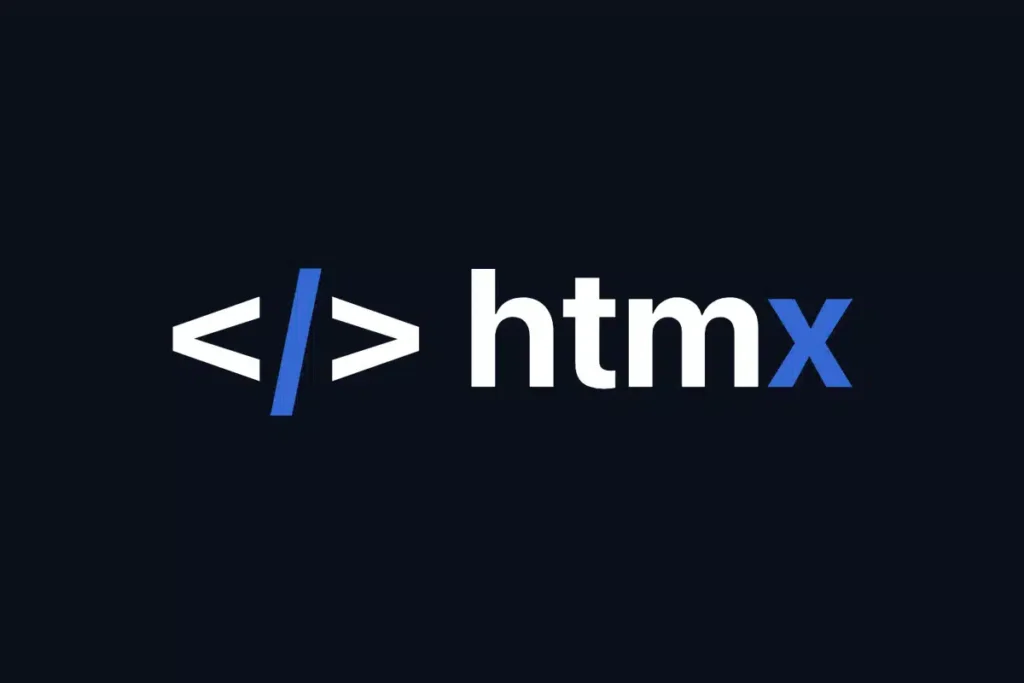
Exploring HTMX: A Lightweight Framework for Dynamic Web Applications
Imagine a framework that provides all the benefits and features of React or Vue.js while retaining the simplicity of HTML. That framework exists, and it’s called HTMX.
HTMX enhances HTML by adding the ability to perform AJAX calls, WebSocket communication, server-sent events, and transitions—all through plain HTML. In this article, we’ll explore the advantages of HTMX, how to integrate it into a web application, and why you might consider replacing your current framework with HTMX.
1. What is HTMX?
HTMX is a framework that allows developers to create interactive and dynamic web pages using only HTML. Unlike other frameworks like React or Vue.js, HTMX does not require you to write complex JavaScript code to handle dynamic behaviors. Instead, it extends HTML with special attributes that enable dynamic actions like making HTTP requests or updating the DOM without reloading the page.
For example, consider the following standard HTML tag:
<a href="/myblog">Blog</a>
Clicking this link triggers an HTTP request to the /myblog
route, which then loads the appropriate HTML content. With HTMX, you can perform HTTP requests from any element in the page, not just links or forms. Here’s how you might enhance the functionality of a button using HTMX:
<button
hx-post="/get-data"
hx-trigger="click"
hx-target="#parent-div"
hx-swap="outerHTML"
>
Click Me!
</button>
When the button is clicked, HTMX sends a POST request to /get-data
, fetches the response, and replaces the content of the element with the ID parent-div
with the response.
HTMX transforms HTML into a powerful language that can:
- Send HTTP requests from any element, not just forms or links.
- Trigger requests from any event, not just clicks or form submissions.
- Use any HTTP method (GET, POST, PUT, DELETE, etc.).
- Update any DOM element with the response of an HTTP request.
2. Advantages of Using HTMX
HTMX offers several key advantages over traditional JavaScript frameworks:
Ease of Learning
If you’re familiar with HTML, you can quickly get up to speed with HTMX. It’s ideal for developers who want to add dynamic behavior to web pages without diving deep into JavaScript frameworks. HTMX’s syntax is intuitive and leverages existing HTML knowledge, making it easy to implement even for those who aren’t JavaScript experts.
Improved Performance
HTMX enables server-side rendering of components, reducing the amount of work performed on the client side. This shift reduces the client’s processing load, resulting in faster load times and smoother user experiences. By eliminating unnecessary JavaScript, HTMX can significantly boost the performance of your web application.
Enhanced Accessibility
HTMX was developed with accessibility in mind. Its focus on simple HTML and clean URL structures ensures that applications built with HTMX are accessible to a wide range of users, including those with disabilities. This is especially important for creating web applications that comply with web accessibility standards.
SEO Benefits
HTMX facilitates clean URLs and semantic HTML, both of which are critical for SEO. By keeping the HTML structure intact and avoiding complex JavaScript rendering on the client side, HTMX ensures that search engines can easily crawl and index your web pages.
These advantages make HTMX an attractive option for developers looking for a lightweight, easy-to-use framework that doesn’t compromise on performance or accessibility.
3. Getting Started with HTMX
Using HTMX is incredibly simple. To start, you need to import the HTMX library by adding the following script tag in the <head>
section of your HTML document:
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://unpkg.com/htmx.org@1.9.2"></script>
</head>
<body></body>
</html>
Once HTMX is included, you can use its attributes on any element inside the <body>
tag to trigger HTTP requests and handle responses.
Setting Up a Simple Server
For our example, we’ll use an Express server to handle the HTTP requests. First, make sure you have Node.js installed, then follow these steps:
- Create an empty directory for your project.
- Open a terminal and navigate to the project directory.
- Initialize a new Node.js project with:
npm init -y
- Install Express by running:
npm install express
Your server is now ready to handle API requests.
4. Making a GET Request with HTMX
Let’s say you want to make a simple GET request from your HTML. You can do this using the hx-get
attribute, which specifies the URL to send the request to:
<button hx-get="http://localhost:5000/hello-world" hx-swap="outerHTML">
Say Hello!
</button>
In this example, clicking the button sends a GET request to the /hello-world
route and replaces the button’s content with the server’s response.
You can also specify where the response should be inserted using the hx-target
attribute:
<button
hx-get="http://localhost:5000/hello-world"
hx-target="#response-div"
hx-swap="outerHTML"
>
Say Hello!
</button>
<div id="response-div"></div>
Now the server’s response will be inserted into the <div>
element with the ID response-div
.
Creating the API Endpoint
To handle the GET request, create a file named server.js
with the following code:
const express = require("express");
const app = express();
app.get("/hello-world", (req, res) => {
res.send("Hello, World!");
});
app.listen(5000, () => {
console.log("Server is running on port 5000");
});
Start the server by running:
node server.js
When you click the button, HTMX will asynchronously fetch the response from the server and insert it into the target element—without reloading the page.
Making POST, PUT, and DELETE Requests
HTMX supports all HTTP methods, not just GET. You can use hx-post
, hx-put
, or hx-delete
to send different types of requests. For example:
<button hx-post="/submit-data">Submit</button>
The hx-target
attribute can use CSS selectors to specify the target element. For instance, hx-target="next #response-div"
will select the next sibling element with the ID response-div
.
5. Configuring Custom Events with HTMX
The hx-trigger
attribute allows you to customize the event that triggers the HTTP request. You can also add modifiers like delay
or throttle
to control the request timing:
<button hx-get="..." hx-target="..." hx-trigger="keyup changed delay:1s">
Key Up Event
</button>
In this case, the GET request will be triggered by the keyup
event, but only after a 1-second delay. While the request is processing, a loading indicator can be displayed using the .htmx-indicator
class:
.htmx-indicator {
opacity: 0;
transition: opacity 500ms ease-in;
}
.htmx-request .htmx-indicator {
opacity: 1;
}
6. Client-Side Routing with HTMX
HTMX includes a client-side routing system called Boost. This allows you to turn a traditional multi-page application into a single-page application (SPA):
<div hx-boost="true">
<a href="/home">Home</a>
<a href="/about">About</a>
</div>
<body>
<!-- The current page content will be dynamically inserted here -->
</body>
Boost behaves similarly to SPA frameworks like React or Vue.js by swapping content within the <body>
tag without full page reloads.
7. HTMX Event System
HTMX offers a powerful event system that lets you intercept events triggered by requests. For example, you can handle a 404 error response and show an alert message like this:
document.body.addEventListener("htmx:beforeSwap", (event) => {
if (event.detail.xhr.status === 404) {
alert("Page not found!");
}
});
You can also attach JavaScript functions to HTML elements using the hx-on
attribute:
<button hx-on="click: alert('Button clicked!')">
Click Me!
</button>
Conclusion
HTMX is a powerful, lightweight framework that enhances HTML by adding dynamic capabilities like AJAX requests and WebSocket communication—all without needing JavaScript frameworks. By simplifying the development process and focusing on server-side rendering, HTMX offers better performance, accessibility, and SEO benefits.
If you’re looking for an alternative to JavaScript-heavy frameworks, HTMX is worth considering for your next project. It allows developers to create interactive web applications with ease, leveraging the simplicity of HTML while providing all the dynamic functionality needed for modern web development.